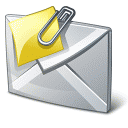
Here is an example to send an email with attachment from your machine.
We assume that mail server is running on localhost and is connected to internet to send email.
For prerequisites please refer to my previous article on JavaMail API.
// File Name SendFileEmail.java import java.util.*; import javax.mail.*; import javax.mail.internet.*; import javax.activation.*; public class SendFileEmail { public static void main(String [] args) { // Recipient's email ID needs to be mentioned. String to = "abcd@gmail.com"; // Sender's email ID needs to be mentioned String from = "web@gmail.com"; // Assuming you are sending email from localhost String host = "localhost"; // Get system properties Properties properties = System.getProperties(); // Setup mail server properties.setProperty("mail.smtp.host", host); // Get the default Session object. Session session = Session.getDefaultInstance(properties); try{ // Create a default MimeMessage object. MimeMessage message = new MimeMessage(session); // Set From: header field of the header. message.setFrom(new InternetAddress(from)); // Set To: header field of the header. message.addRecipient(Message.RecipientType.TO, new InternetAddress(to)); // Set Subject: header field message.setSubject("This is the Subject Line!"); // Create the message part BodyPart messageBodyPart = new MimeBodyPart(); // Fill the message messageBodyPart.setText("This is message body"); // Create a multipar message Multipart multipart = new MimeMultipart(); // Set text message part multipart.addBodyPart(messageBodyPart); // Part two is attachment messageBodyPart = new MimeBodyPart(); String filename = "file.txt"; DataSource source = new FileDataSource(filename); messageBodyPart.setDataHandler(new DataHandler(source)); messageBodyPart.setFileName(filename); multipart.addBodyPart(messageBodyPart); // Send the complete message parts message.setContent(multipart ); // Send message Transport.send(message); System.out.println("Sent message successfully...."); }catch (MessagingException mex) { mex.printStackTrace(); } } }
Compile and run this program to send an HTML email:
$ java SendFileEmail Sent message successfully....
User Authentication
If it is required to provide user ID and Password to the email server for authentication purpose then you can set these properties as follows:
props.setProperty("mail.user", "myuser"); props.setProperty("mail.password", "mypwd");Rest of the email sending mechanism would remain as explained above.
No comments:
Post a Comment